Prompts
简介
Laravel Prompts 是一个 PHP 软件包,用于向您的命令行应用程序添加美观且用户友好的表单,具有类似浏览器的功能,包括占位符文本和验证。
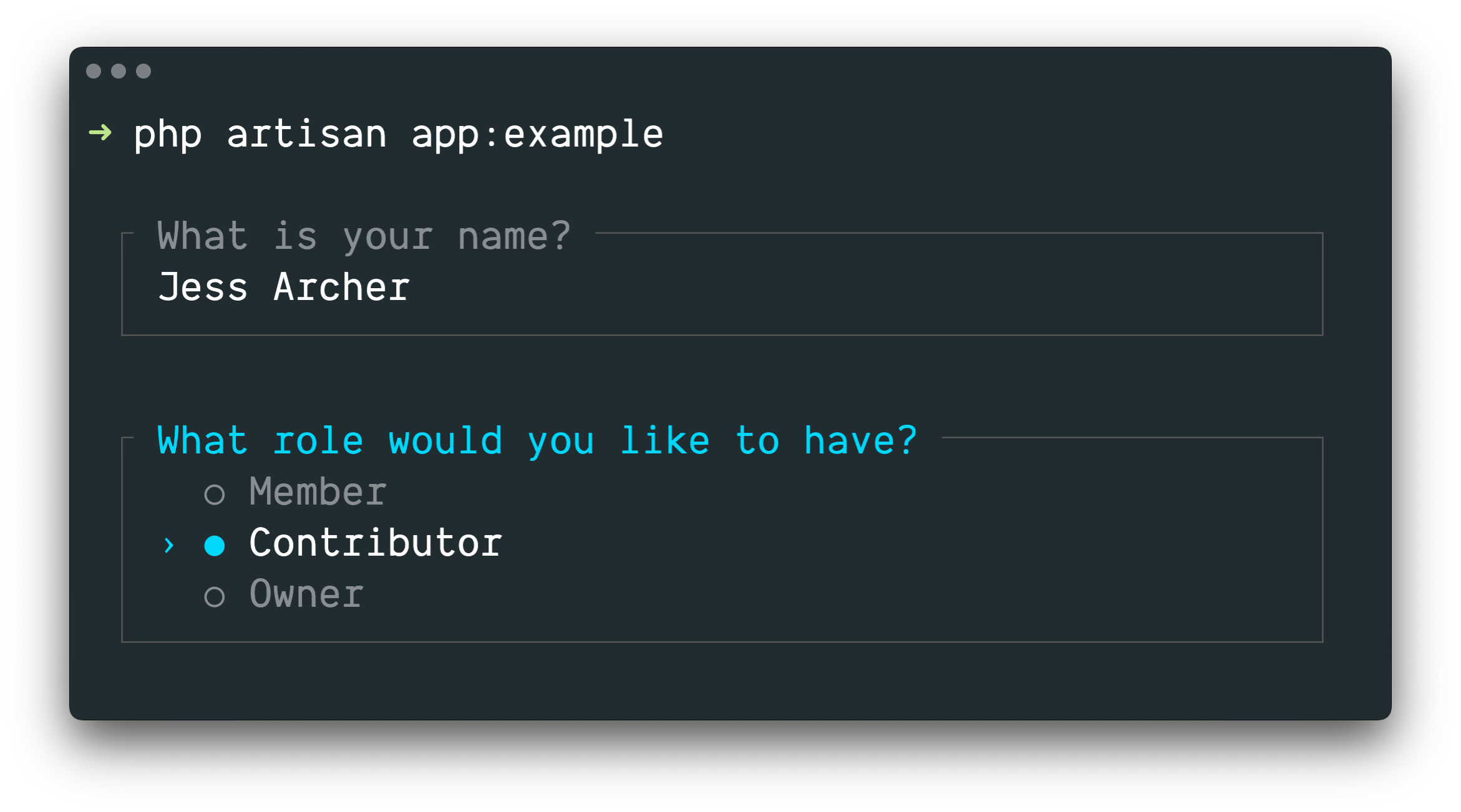
Laravel Prompts 非常适合在您的 Artisan 控制台命令中接受用户输入,但它也可以用于任何命令行 PHP 项目。
Laravel Prompts 支持 macOS、Linux 和 Windows with WSL。有关更多信息,请参阅我们关于不支持的环境和回退的文档。
安装
Laravel Prompts 已经包含在最新版本的 Laravel 中。
Laravel Prompts 也可以使用 Composer 包管理器安装在您的其他 PHP 项目中
1composer require laravel/prompts
可用的 Prompts
文本
text
函数将使用给定的问题提示用户,接受他们的输入,然后返回它
1use function Laravel\Prompts\text;2 3$name = text('What is your name?');
您还可以包含占位符文本、默认值和信息提示
1$name = text(2 label: 'What is your name?',3 placeholder: 'E.g. Taylor Otwell',4 default: $user?->name,5 hint: 'This will be displayed on your profile.'6);
必填值
如果您要求必须输入值,您可以传递 required
参数
1$name = text(2 label: 'What is your name?',3 required: true4);
如果您想自定义验证消息,您也可以传递一个字符串
1$name = text(2 label: 'What is your name?',3 required: 'Your name is required.'4);
其他验证
最后,如果您想执行其他验证逻辑,您可以将闭包传递给 validate
参数
1$name = text(2 label: 'What is your name?',3 validate: fn (string $value) => match (true) {4 strlen($value) < 3 => 'The name must be at least 3 characters.',5 strlen($value) > 255 => 'The name must not exceed 255 characters.',6 default => null7 }8);
闭包将接收已输入的值,并且可以返回错误消息,或者在验证通过时返回 null
。
或者,您可以利用 Laravel 验证器的强大功能。为此,请向 validate
参数提供一个数组,其中包含属性名称和所需的验证规则
1$name = text(2 label: 'What is your name?',3 validate: ['name' => 'required|max:255|unique:users']4);
文本区域
textarea
函数将使用给定的问题提示用户,通过多行文本区域接受他们的输入,然后返回它
1use function Laravel\Prompts\textarea;2 3$story = textarea('Tell me a story.');
您还可以包含占位符文本、默认值和信息提示
1$story = textarea(2 label: 'Tell me a story.',3 placeholder: 'This is a story about...',4 hint: 'This will be displayed on your profile.'5);
必填值
如果您要求必须输入值,您可以传递 required
参数
1$story = textarea(2 label: 'Tell me a story.',3 required: true4);
如果您想自定义验证消息,您也可以传递一个字符串
1$story = textarea(2 label: 'Tell me a story.',3 required: 'A story is required.'4);
其他验证
最后,如果您想执行其他验证逻辑,您可以将闭包传递给 validate
参数
1$story = textarea(2 label: 'Tell me a story.',3 validate: fn (string $value) => match (true) {4 strlen($value) < 250 => 'The story must be at least 250 characters.',5 strlen($value) > 10000 => 'The story must not exceed 10,000 characters.',6 default => null7 }8);
闭包将接收已输入的值,并且可以返回错误消息,或者在验证通过时返回 null
。
或者,您可以利用 Laravel 验证器的强大功能。为此,请向 validate
参数提供一个数组,其中包含属性名称和所需的验证规则
1$story = textarea(2 label: 'Tell me a story.',3 validate: ['story' => 'required|max:10000']4);
密码
password
函数类似于 text
函数,但用户的输入在控制台中输入时将被屏蔽。这在询问密码等敏感信息时非常有用
1use function Laravel\Prompts\password;2 3$password = password('What is your password?');
您还可以包含占位符文本和信息提示
1$password = password(2 label: 'What is your password?',3 placeholder: 'password',4 hint: 'Minimum 8 characters.'5);
必填值
如果您要求必须输入值,您可以传递 required
参数
1$password = password(2 label: 'What is your password?',3 required: true4);
如果您想自定义验证消息,您也可以传递一个字符串
1$password = password(2 label: 'What is your password?',3 required: 'The password is required.'4);
其他验证
最后,如果您想执行其他验证逻辑,您可以将闭包传递给 validate
参数
1$password = password(2 label: 'What is your password?',3 validate: fn (string $value) => match (true) {4 strlen($value) < 8 => 'The password must be at least 8 characters.',5 default => null6 }7);
闭包将接收已输入的值,并且可以返回错误消息,或者在验证通过时返回 null
。
或者,您可以利用 Laravel 验证器的强大功能。为此,请向 validate
参数提供一个数组,其中包含属性名称和所需的验证规则
1$password = password(2 label: 'What is your password?',3 validate: ['password' => 'min:8']4);
确认
如果您需要询问用户“是或否”确认,您可以使用 confirm
函数。用户可以使用箭头键或按 y
或 n
来选择他们的响应。此函数将返回 true
或 false
。
1use function Laravel\Prompts\confirm;2 3$confirmed = confirm('Do you accept the terms?');
您还可以包含默认值、自定义的“是”和“否”标签措辞以及信息提示
1$confirmed = confirm(2 label: 'Do you accept the terms?',3 default: false,4 yes: 'I accept',5 no: 'I decline',6 hint: 'The terms must be accepted to continue.'7);
要求“是”
如有必要,您可以要求用户通过传递 required
参数来选择“是”
1$confirmed = confirm(2 label: 'Do you accept the terms?',3 required: true4);
如果您想自定义验证消息,您也可以传递一个字符串
1$confirmed = confirm(2 label: 'Do you accept the terms?',3 required: 'You must accept the terms to continue.'4);
选择
如果您需要用户从预定义的选择集中进行选择,您可以使用 select
函数
1use function Laravel\Prompts\select;2 3$role = select(4 label: 'What role should the user have?',5 options: ['Member', 'Contributor', 'Owner']6);
您还可以指定默认选项和信息提示
1$role = select(2 label: 'What role should the user have?',3 options: ['Member', 'Contributor', 'Owner'],4 default: 'Owner',5 hint: 'The role may be changed at any time.'6);
您还可以将关联数组传递给 options
参数,以便返回选定的键而不是其值
1$role = select(2 label: 'What role should the user have?',3 options: [4 'member' => 'Member',5 'contributor' => 'Contributor',6 'owner' => 'Owner',7 ],8 default: 'owner'9);
在列表开始滚动之前,最多会显示五个选项。您可以通过传递 scroll
参数来自定义此设置
1$role = select(2 label: 'Which category would you like to assign?',3 options: Category::pluck('name', 'id'),4 scroll: 105);
其他验证
与其他 prompt 函数不同,select
函数不接受 required
参数,因为它不可能什么都不选择。但是,如果您需要呈现一个选项但阻止其被选中,您可以将闭包传递给 validate
参数
1$role = select( 2 label: 'What role should the user have?', 3 options: [ 4 'member' => 'Member', 5 'contributor' => 'Contributor', 6 'owner' => 'Owner', 7 ], 8 validate: fn (string $value) => 9 $value === 'owner' && User::where('role', 'owner')->exists()10 ? 'An owner already exists.'11 : null12);
如果 options
参数是关联数组,则闭包将接收选定的键,否则将接收选定的值。闭包可以返回错误消息,或者在验证通过时返回 null
。
多选
如果您需要用户能够选择多个选项,您可以使用 multiselect
函数
1use function Laravel\Prompts\multiselect;2 3$permissions = multiselect(4 label: 'What permissions should be assigned?',5 options: ['Read', 'Create', 'Update', 'Delete']6);
您还可以指定默认选择和信息提示
1use function Laravel\Prompts\multiselect;2 3$permissions = multiselect(4 label: 'What permissions should be assigned?',5 options: ['Read', 'Create', 'Update', 'Delete'],6 default: ['Read', 'Create'],7 hint: 'Permissions may be updated at any time.'8);
您还可以将关联数组传递给 options
参数,以返回所选选项的键而不是它们的值
1$permissions = multiselect( 2 label: 'What permissions should be assigned?', 3 options: [ 4 'read' => 'Read', 5 'create' => 'Create', 6 'update' => 'Update', 7 'delete' => 'Delete', 8 ], 9 default: ['read', 'create']10);
在列表开始滚动之前,最多会显示五个选项。您可以通过传递 scroll
参数来自定义此设置
1$categories = multiselect(2 label: 'What categories should be assigned?',3 options: Category::pluck('name', 'id'),4 scroll: 105);
要求值
默认情况下,用户可以选择零个或多个选项。您可以传递 required
参数来强制要求一个或多个选项
1$categories = multiselect(2 label: 'What categories should be assigned?',3 options: Category::pluck('name', 'id'),4 required: true5);
如果您想自定义验证消息,您可以向 required
参数提供一个字符串
1$categories = multiselect(2 label: 'What categories should be assigned?',3 options: Category::pluck('name', 'id'),4 required: 'You must select at least one category'5);
其他验证
如果您需要呈现一个选项但阻止其被选中,您可以将闭包传递给 validate
参数
1$permissions = multiselect( 2 label: 'What permissions should the user have?', 3 options: [ 4 'read' => 'Read', 5 'create' => 'Create', 6 'update' => 'Update', 7 'delete' => 'Delete', 8 ], 9 validate: fn (array $values) => ! in_array('read', $values)10 ? 'All users require the read permission.'11 : null12);
如果 options
参数是关联数组,则闭包将接收选定的键,否则将接收选定的值。闭包可以返回错误消息,或者在验证通过时返回 null
。
建议
suggest
函数可用于为可能的选择提供自动完成功能。用户仍然可以提供任何答案,而无需考虑自动完成提示
1use function Laravel\Prompts\suggest;2 3$name = suggest('What is your name?', ['Taylor', 'Dayle']);
或者,您可以将闭包作为第二个参数传递给 suggest
函数。每次用户键入输入字符时都会调用闭包。闭包应接受一个字符串参数,其中包含用户到目前为止的输入,并返回一个用于自动完成的选项数组
1$name = suggest(2 label: 'What is your name?',3 options: fn ($value) => collect(['Taylor', 'Dayle'])4 ->filter(fn ($name) => Str::contains($name, $value, ignoreCase: true))5)
您还可以包含占位符文本、默认值和信息提示
1$name = suggest(2 label: 'What is your name?',3 options: ['Taylor', 'Dayle'],4 placeholder: 'E.g. Taylor',5 default: $user?->name,6 hint: 'This will be displayed on your profile.'7);
必填值
如果您要求必须输入值,您可以传递 required
参数
1$name = suggest(2 label: 'What is your name?',3 options: ['Taylor', 'Dayle'],4 required: true5);
如果您想自定义验证消息,您也可以传递一个字符串
1$name = suggest(2 label: 'What is your name?',3 options: ['Taylor', 'Dayle'],4 required: 'Your name is required.'5);
其他验证
最后,如果您想执行其他验证逻辑,您可以将闭包传递给 validate
参数
1$name = suggest(2 label: 'What is your name?',3 options: ['Taylor', 'Dayle'],4 validate: fn (string $value) => match (true) {5 strlen($value) < 3 => 'The name must be at least 3 characters.',6 strlen($value) > 255 => 'The name must not exceed 255 characters.',7 default => null8 }9);
闭包将接收已输入的值,并且可以返回错误消息,或者在验证通过时返回 null
。
或者,您可以利用 Laravel 验证器的强大功能。为此,请向 validate
参数提供一个数组,其中包含属性名称和所需的验证规则
1$name = suggest(2 label: 'What is your name?',3 options: ['Taylor', 'Dayle'],4 validate: ['name' => 'required|min:3|max:255']5);
搜索
如果用户有很多选项可供选择,则 search
函数允许用户键入搜索查询以过滤结果,然后再使用箭头键选择选项
1use function Laravel\Prompts\search;2 3$id = search(4 label: 'Search for the user that should receive the mail',5 options: fn (string $value) => strlen($value) > 06 ? User::whereLike('name', "%{$value}%")->pluck('name', 'id')->all()7 : []8);
闭包将接收用户到目前为止键入的文本,并且必须返回一个选项数组。如果您返回关联数组,则将返回所选选项的键,否则将返回其值。
当过滤您打算返回值的数组时,您应该使用 array_values
函数或 values
Collection 方法来确保数组不会变成关联数组
1$names = collect(['Taylor', 'Abigail']);2 3$selected = search(4 label: 'Search for the user that should receive the mail',5 options: fn (string $value) => $names6 ->filter(fn ($name) => Str::contains($name, $value, ignoreCase: true))7 ->values()8 ->all(),9);
您还可以包含占位符文本和信息提示
1$id = search(2 label: 'Search for the user that should receive the mail',3 placeholder: 'E.g. Taylor Otwell',4 options: fn (string $value) => strlen($value) > 05 ? User::whereLike('name', "%{$value}%")->pluck('name', 'id')->all()6 : [],7 hint: 'The user will receive an email immediately.'8);
在列表开始滚动之前,最多会显示五个选项。您可以通过传递 scroll
参数来自定义此设置
1$id = search(2 label: 'Search for the user that should receive the mail',3 options: fn (string $value) => strlen($value) > 04 ? User::whereLike('name', "%{$value}%")->pluck('name', 'id')->all()5 : [],6 scroll: 107);
其他验证
如果您想执行其他验证逻辑,您可以将闭包传递给 validate
参数
1$id = search( 2 label: 'Search for the user that should receive the mail', 3 options: fn (string $value) => strlen($value) > 0 4 ? User::whereLike('name', "%{$value}%")->pluck('name', 'id')->all() 5 : [], 6 validate: function (int|string $value) { 7 $user = User::findOrFail($value); 8 9 if ($user->opted_out) {10 return 'This user has opted-out of receiving mail.';11 }12 }13);
如果 options
闭包返回关联数组,则闭包将接收选定的键,否则,它将接收选定的值。闭包可以返回错误消息,或者在验证通过时返回 null
。
多重搜索
如果您有很多可搜索的选项,并且需要用户能够选择多个项目,则 multisearch
函数允许用户键入搜索查询以过滤结果,然后再使用箭头键和空格键选择选项
1use function Laravel\Prompts\multisearch;2 3$ids = multisearch(4 'Search for the users that should receive the mail',5 fn (string $value) => strlen($value) > 06 ? User::whereLike('name', "%{$value}%")->pluck('name', 'id')->all()7 : []8);
闭包将接收用户到目前为止键入的文本,并且必须返回一个选项数组。如果您返回关联数组,则将返回所选选项的键;否则,将返回它们的值。
当过滤您打算返回值的数组时,您应该使用 array_values
函数或 values
Collection 方法来确保数组不会变成关联数组
1$names = collect(['Taylor', 'Abigail']);2 3$selected = multisearch(4 label: 'Search for the users that should receive the mail',5 options: fn (string $value) => $names6 ->filter(fn ($name) => Str::contains($name, $value, ignoreCase: true))7 ->values()8 ->all(),9);
您还可以包含占位符文本和信息提示
1$ids = multisearch(2 label: 'Search for the users that should receive the mail',3 placeholder: 'E.g. Taylor Otwell',4 options: fn (string $value) => strlen($value) > 05 ? User::whereLike('name', "%{$value}%")->pluck('name', 'id')->all()6 : [],7 hint: 'The user will receive an email immediately.'8);
在列表开始滚动之前,最多会显示五个选项。您可以通过提供 scroll
参数来自定义此设置
1$ids = multisearch(2 label: 'Search for the users that should receive the mail',3 options: fn (string $value) => strlen($value) > 04 ? User::whereLike('name', "%{$value}%")->pluck('name', 'id')->all()5 : [],6 scroll: 107);
要求值
默认情况下,用户可以选择零个或多个选项。您可以传递 required
参数来强制要求一个或多个选项
1$ids = multisearch(2 label: 'Search for the users that should receive the mail',3 options: fn (string $value) => strlen($value) > 04 ? User::whereLike('name', "%{$value}%")->pluck('name', 'id')->all()5 : [],6 required: true7);
如果您想自定义验证消息,您也可以向 required
参数提供一个字符串
1$ids = multisearch(2 label: 'Search for the users that should receive the mail',3 options: fn (string $value) => strlen($value) > 04 ? User::whereLike('name', "%{$value}%")->pluck('name', 'id')->all()5 : [],6 required: 'You must select at least one user.'7);
其他验证
如果您想执行其他验证逻辑,您可以将闭包传递给 validate
参数
1$ids = multisearch( 2 label: 'Search for the users that should receive the mail', 3 options: fn (string $value) => strlen($value) > 0 4 ? User::whereLike('name', "%{$value}%")->pluck('name', 'id')->all() 5 : [], 6 validate: function (array $values) { 7 $optedOut = User::whereLike('name', '%a%')->findMany($values); 8 9 if ($optedOut->isNotEmpty()) {10 return $optedOut->pluck('name')->join(', ', ', and ').' have opted out.';11 }12 }13);
如果 options
闭包返回关联数组,则闭包将接收选定的键;否则,它将接收选定的值。闭包可以返回错误消息,或者在验证通过时返回 null
。
暂停
pause
函数可用于向用户显示信息性文本,并等待他们按下 Enter / Return 键以确认他们想要继续操作
1use function Laravel\Prompts\pause;2 3pause('Press ENTER to continue.');
验证前转换输入
有时您可能希望在进行验证之前转换 prompt 输入。例如,您可能希望从任何提供的字符串中删除空格。为了实现这一点,许多 prompt 函数都提供了 transform
参数,该参数接受闭包
1$name = text(2 label: 'What is your name?',3 transform: fn (string $value) => trim($value),4 validate: fn (string $value) => match (true) {5 strlen($value) < 3 => 'The name must be at least 3 characters.',6 strlen($value) > 255 => 'The name must not exceed 255 characters.',7 default => null8 }9);
表单
通常,您会有多个 prompt 按顺序显示以收集信息,然后再执行其他操作。您可以使用 form
函数创建一组分组的 prompt,供用户完成
1use function Laravel\Prompts\form;2 3$responses = form()4 ->text('What is your name?', required: true)5 ->password('What is your password?', validate: ['password' => 'min:8'])6 ->confirm('Do you accept the terms?')7 ->submit();
submit
方法将返回一个数字索引数组,其中包含表单 prompt 的所有响应。但是,您可以通过 name
参数为每个 prompt 提供名称。当提供名称时,可以通过该名称访问命名的 prompt 的响应
1use App\Models\User; 2use function Laravel\Prompts\form; 3 4$responses = form() 5 ->text('What is your name?', required: true, name: 'name') 6 ->password( 7 label: 'What is your password?', 8 validate: ['password' => 'min:8'], 9 name: 'password'10 )11 ->confirm('Do you accept the terms?')12 ->submit();13 14User::create([15 'name' => $responses['name'],16 'password' => $responses['password'],17]);
使用 form
函数的主要好处是用户可以使用 CTRL + U
返回表单中的先前 prompt。这允许用户修复错误或更改选择,而无需取消并重新启动整个表单。
如果您需要更精细地控制表单中的 prompt,您可以调用 add
方法而不是直接调用 prompt 函数之一。add
方法会传递用户提供的所有先前响应
1use function Laravel\Prompts\form; 2use function Laravel\Prompts\outro; 3 4$responses = form() 5 ->text('What is your name?', required: true, name: 'name') 6 ->add(function ($responses) { 7 return text("How old are you, {$responses['name']}?"); 8 }, name: 'age') 9 ->submit();10 11outro("Your name is {$responses['name']} and you are {$responses['age']} years old.");
信息性消息
note
、info
、warning
、error
和 alert
函数可用于显示信息性消息
1use function Laravel\Prompts\info;2 3info('Package installed successfully.');
表格
table
函数使显示多行和多列数据变得容易。您只需要提供列名和表格数据即可
1use function Laravel\Prompts\table;2 3table(4 headers: ['Name', 'Email'],5 rows: User::all(['name', 'email'])->toArray()6);
Spin
spin
函数在执行指定的回调时显示一个微调器以及可选消息。它用于指示正在进行的过程,并在完成时返回回调的结果
1use function Laravel\Prompts\spin;2 3$response = spin(4 message: 'Fetching response...',5 callback: fn () => Http::get('http://example.com')6);
spin
函数需要 pcntl
PHP 扩展来动画微调器。当此扩展不可用时,将显示微调器的静态版本。
进度条
对于长时间运行的任务,显示进度条以告知用户任务的完成程度可能很有帮助。使用 progress
函数,Laravel 将显示一个进度条,并为给定可迭代值的每次迭代推进其进度
1use function Laravel\Prompts\progress;2 3$users = progress(4 label: 'Updating users',5 steps: User::all(),6 callback: fn ($user) => $this->performTask($user)7);
progress
函数的作用类似于 map 函数,并将返回一个数组,其中包含回调的每次迭代的返回值。
回调也可以接受 Laravel\Prompts\Progress
实例,允许您在每次迭代时修改标签和提示
1$users = progress( 2 label: 'Updating users', 3 steps: User::all(), 4 callback: function ($user, $progress) { 5 $progress 6 ->label("Updating {$user->name}") 7 ->hint("Created on {$user->created_at}"); 8 9 return $this->performTask($user);10 },11 hint: 'This may take some time.'12);
有时,您可能需要更手动地控制进度条的推进方式。首先,定义进程将迭代的总步数。然后,在处理完每个项目后,通过 advance
方法推进进度条
1$progress = progress(label: 'Updating users', steps: 10); 2 3$users = User::all(); 4 5$progress->start(); 6 7foreach ($users as $user) { 8 $this->performTask($user); 9 10 $progress->advance();11}12 13$progress->finish();
清除终端
clear
函数可用于清除用户的终端
1use function Laravel\Prompts\clear;2 3clear();
终端注意事项
终端宽度
如果任何标签、选项或验证消息的长度超过用户终端中的“列”数,它将自动截断以适应。如果您的用户可能正在使用较窄的终端,请考虑最小化这些字符串的长度。通常,为了支持 80 个字符的终端,安全的最大长度为 74 个字符。
终端高度
对于任何接受 scroll
参数的 prompt,配置的值将自动减少以适应用户终端的高度,包括验证消息的空间。
不支持的环境和回退
Laravel Prompts 支持 macOS、Linux 和 Windows with WSL。由于 Windows 版本 PHP 的限制,目前无法在 WSL 之外的 Windows 上使用 Laravel Prompts。
因此,Laravel Prompts 支持回退到替代实现,例如 Symfony Console Question Helper。
当将 Laravel Prompts 与 Laravel 框架一起使用时,已为您配置了每个 prompt 的回退,并且将在不支持的环境中自动启用。
回退条件
如果您未使用 Laravel 或需要自定义何时使用回退行为,您可以将布尔值传递给 Prompt
类上的 fallbackWhen
静态方法
1use Laravel\Prompts\Prompt;2 3Prompt::fallbackWhen(4 ! $input->isInteractive() || windows_os() || app()->runningUnitTests()5);
回退行为
如果您未使用 Laravel 或需要自定义回退行为,您可以将闭包传递给每个 prompt 类上的 fallbackUsing
静态方法
1use Laravel\Prompts\TextPrompt; 2use Symfony\Component\Console\Question\Question; 3use Symfony\Component\Console\Style\SymfonyStyle; 4 5TextPrompt::fallbackUsing(function (TextPrompt $prompt) use ($input, $output) { 6 $question = (new Question($prompt->label, $prompt->default ?: null)) 7 ->setValidator(function ($answer) use ($prompt) { 8 if ($prompt->required && $answer === null) { 9 throw new \RuntimeException(10 is_string($prompt->required) ? $prompt->required : 'Required.'11 );12 }13 14 if ($prompt->validate) {15 $error = ($prompt->validate)($answer ?? '');16 17 if ($error) {18 throw new \RuntimeException($error);19 }20 }21 22 return $answer;23 });24 25 return (new SymfonyStyle($input, $output))26 ->askQuestion($question);27});
必须为每个 prompt 类单独配置回退。闭包将接收 prompt 类的实例,并且必须为 prompt 返回适当的类型。