Laravel Telescope
简介
Laravel Telescope 是您本地 Laravel 开发环境的绝佳伴侣。 Telescope 提供了对进入您应用程序的请求、异常、日志条目、数据库查询、队列任务、邮件、通知、缓存操作、计划任务、变量转储等的深入了解。
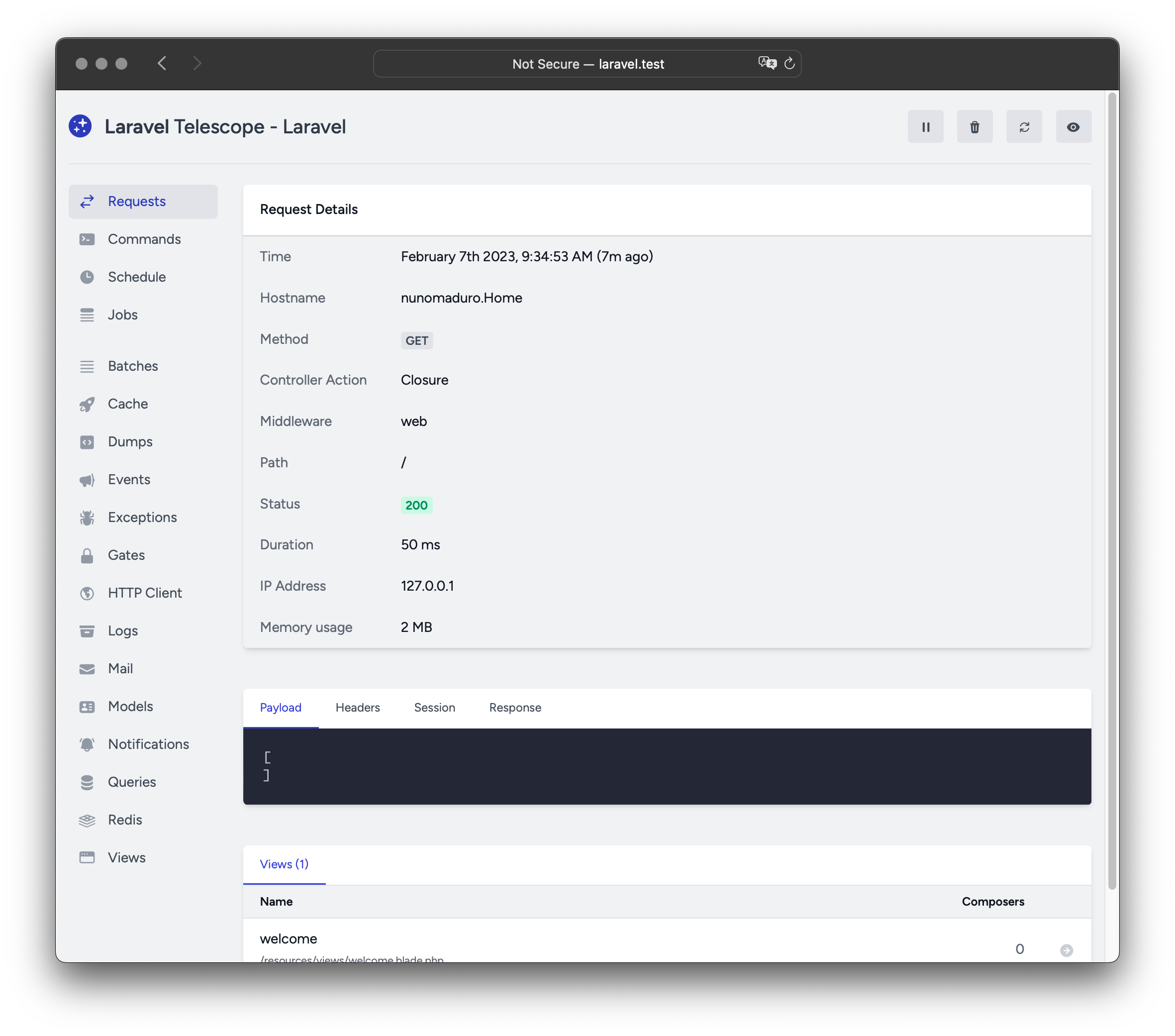
安装
您可以使用 Composer 包管理器将 Telescope 安装到您的 Laravel 项目中
1composer require laravel/telescope
安装 Telescope 后,使用 telescope:install
Artisan 命令发布其资源和迁移。 安装 Telescope 后,您还应该运行 migrate
命令以创建存储 Telescope 数据所需的表。
1php artisan telescope:install2 3php artisan migrate
最后,您可以通过 /telescope
路由访问 Telescope 仪表盘。
仅本地安装
如果您计划仅使用 Telescope 来辅助本地开发,则可以使用 --dev
标志安装 Telescope
1composer require laravel/telescope --dev2 3php artisan telescope:install4 5php artisan migrate
运行 telescope:install
后,您应该从应用程序的 bootstrap/providers.php
配置文件中删除 TelescopeServiceProvider
服务提供者的注册。 相反,在 App\Providers\AppServiceProvider
类的 register
方法中手动注册 Telescope 的服务提供者。 我们将确保当前环境为 local
,然后再注册提供者
1/** 2 * Register any application services. 3 */ 4public function register(): void 5{ 6 if ($this->app->environment('local') && class_exists(\Laravel\Telescope\TelescopeServiceProvider::class)) { 7 $this->app->register(\Laravel\Telescope\TelescopeServiceProvider::class); 8 $this->app->register(TelescopeServiceProvider::class); 9 }10}
最后,您还应该通过将以下内容添加到您的 composer.json
文件中来阻止 Telescope 包被自动发现
1"extra": {2 "laravel": {3 "dont-discover": [4 "laravel/telescope"5 ]6 }7},
配置
发布 Telescope 的资源后,其主要配置文件将位于 config/telescope.php
。 此配置文件允许您配置监视器选项。 每个配置选项都包含对其用途的描述,因此请务必彻底浏览此文件。
如果需要,您可以完全禁用 Telescope 的数据收集,使用 enabled
配置选项
1'enabled' => env('TELESCOPE_ENABLED', true),
数据剪枝
如果不进行剪枝,telescope_entries
表可能会非常快速地累积记录。 为了缓解这种情况,您应该计划每天运行 telescope:prune
Artisan 命令
1use Illuminate\Support\Facades\Schedule;2 3Schedule::command('telescope:prune')->daily();
默认情况下,所有超过 24 小时的条目都将被剪枝。 您可以在调用命令时使用 hours
选项来确定保留 Telescope 数据的时间。 例如,以下命令将删除所有在 48 小时前创建的记录
1use Illuminate\Support\Facades\Schedule;2 3Schedule::command('telescope:prune --hours=48')->daily();
仪表盘授权
可以通过 /telescope
路由访问 Telescope 仪表盘。 默认情况下,您只能在 local
环境中访问此仪表盘。 在您的 app/Providers/TelescopeServiceProvider.php
文件中,有一个授权门面定义。 此授权门面控制对 非本地 环境中 Telescope 的访问。 您可以根据需要自由修改此门面,以限制对 Telescope 安装的访问
1use App\Models\User; 2 3/** 4 * Register the Telescope gate. 5 * 6 * This gate determines who can access Telescope in non-local environments. 7 */ 8protected function gate(): void 9{10 Gate::define('viewTelescope', function (User $user) {11 return in_array($user->email, [13 ]);14 });15}
您应确保在生产环境中将 APP_ENV
环境变量更改为 production
。 否则,您的 Telescope 安装将公开可用。
升级 Telescope
当升级到 Telescope 的新主要版本时,仔细查看升级指南非常重要。
此外,当升级到任何新的 Telescope 版本时,您都应该重新发布 Telescope 的资源
1php artisan telescope:publish
为了保持资源更新并避免将来更新出现问题,您可以将 vendor:publish --tag=laravel-assets
命令添加到应用程序的 composer.json
文件中的 post-update-cmd
脚本中
1{2 "scripts": {3 "post-update-cmd": [4 "@php artisan vendor:publish --tag=laravel-assets --ansi --force"5 ]6 }7}
过滤
条目
您可以通过在 App\Providers\TelescopeServiceProvider
类中定义的 filter
闭包来过滤 Telescope 记录的数据。 默认情况下,此闭包记录 local
环境中的所有数据,以及所有其他环境中的异常、失败的任务、计划任务和带有监控标签的数据
1use Laravel\Telescope\IncomingEntry; 2use Laravel\Telescope\Telescope; 3 4/** 5 * Register any application services. 6 */ 7public function register(): void 8{ 9 $this->hideSensitiveRequestDetails();10 11 Telescope::filter(function (IncomingEntry $entry) {12 if ($this->app->environment('local')) {13 return true;14 }15 16 return $entry->isReportableException() ||17 $entry->isFailedJob() ||18 $entry->isScheduledTask() ||19 $entry->isSlowQuery() ||20 $entry->hasMonitoredTag();21 });22}
批处理
虽然 filter
闭包会过滤单个条目的数据,但您可以使用 filterBatch
方法注册一个闭包,该闭包会过滤给定请求或控制台命令的所有数据。 如果闭包返回 true
,则所有条目都将由 Telescope 记录
1use Illuminate\Support\Collection; 2use Laravel\Telescope\IncomingEntry; 3use Laravel\Telescope\Telescope; 4 5/** 6 * Register any application services. 7 */ 8public function register(): void 9{10 $this->hideSensitiveRequestDetails();11 12 Telescope::filterBatch(function (Collection $entries) {13 if ($this->app->environment('local')) {14 return true;15 }16 17 return $entries->contains(function (IncomingEntry $entry) {18 return $entry->isReportableException() ||19 $entry->isFailedJob() ||20 $entry->isScheduledTask() ||21 $entry->isSlowQuery() ||22 $entry->hasMonitoredTag();23 });24 });25}
标签
Telescope 允许您按“标签”搜索条目。 通常,标签是 Telescope 自动添加到条目的 Eloquent 模型类名或已验证的用户 ID。 有时,您可能希望将自己的自定义标签附加到条目。 为了实现这一点,您可以使用 Telescope::tag
方法。 tag
方法接受一个应返回标签数组的闭包。 闭包返回的标签将与 Telescope 自动附加到条目的任何标签合并。 通常,您应该在 App\Providers\TelescopeServiceProvider
类的 register
方法中调用 tag
方法
1use Laravel\Telescope\IncomingEntry; 2use Laravel\Telescope\Telescope; 3 4/** 5 * Register any application services. 6 */ 7public function register(): void 8{ 9 $this->hideSensitiveRequestDetails();10 11 Telescope::tag(function (IncomingEntry $entry) {12 return $entry->type === 'request'13 ? ['status:'.$entry->content['response_status']]14 : [];15 });16}
可用监视器
当执行请求或控制台命令时,Telescope “监视器”会收集应用程序数据。 您可以在 config/telescope.php
配置文件中自定义您想要启用的监视器列表
1'watchers' => [2 Watchers\CacheWatcher::class => true,3 Watchers\CommandWatcher::class => true,4 // ...5],
某些监视器还允许您提供其他自定义选项
1'watchers' => [2 Watchers\QueryWatcher::class => [3 'enabled' => env('TELESCOPE_QUERY_WATCHER', true),4 'slow' => 100,5 ],6 // ...7],
批处理监视器
批处理监视器记录有关队列批处理的信息,包括任务和连接信息。
缓存监视器
缓存监视器记录缓存键何时命中、未命中、更新和遗忘的数据。
命令监视器
命令监视器记录每次执行 Artisan 命令时的参数、选项、退出代码和输出。 如果您想排除某些命令不被监视器记录,您可以在 config/telescope.php
文件中的 ignore
选项中指定该命令
1'watchers' => [2 Watchers\CommandWatcher::class => [3 'enabled' => env('TELESCOPE_COMMAND_WATCHER', true),4 'ignore' => ['key:generate'],5 ],6 // ...7],
Dump 监视器
Dump 监视器记录并在 Telescope 中显示您的变量转储。 使用 Laravel 时,可以使用全局 dump
函数转储变量。 Dump 监视器选项卡必须在浏览器中打开才能记录转储,否则,转储将被监视器忽略。
事件监视器
事件监视器记录应用程序调度的任何事件的有效负载、监听器和广播数据。 Laravel 框架的内部事件将被事件监视器忽略。
异常监视器
异常监视器记录应用程序抛出的任何可报告异常的数据和堆栈跟踪。
Gate 监视器
Gate 监视器记录应用程序门面和策略检查的数据和结果。 如果您想排除某些能力不被监视器记录,您可以在 config/telescope.php
文件中的 ignore_abilities
选项中指定这些能力
1'watchers' => [2 Watchers\GateWatcher::class => [3 'enabled' => env('TELESCOPE_GATE_WATCHER', true),4 'ignore_abilities' => ['viewNova'],5 ],6 // ...7],
HTTP 客户端监视器
HTTP 客户端监视器记录应用程序发出的传出HTTP 客户端请求。
Job 监视器
Job 监视器记录应用程序调度的任何任务的数据和状态。
日志监视器
日志监视器记录应用程序写入的任何日志的日志数据。
默认情况下,Telescope 将仅记录 error
级别及以上的日志。 但是,您可以修改应用程序 config/telescope.php
配置文件中的 level
选项来修改此行为
1'watchers' => [2 Watchers\LogWatcher::class => [3 'enabled' => env('TELESCOPE_LOG_WATCHER', true),4 'level' => 'debug',5 ],6 7 // ...8],
邮件监视器
邮件监视器允许您查看应用程序发送的电子邮件的浏览器内预览及其关联数据。 您还可以将电子邮件下载为 .eml
文件。
模型监视器
每当 Eloquent 模型事件被调度时,模型监视器都会记录模型更改。 您可以通过监视器的 events
选项指定应记录哪些模型事件
1'watchers' => [2 Watchers\ModelWatcher::class => [3 'enabled' => env('TELESCOPE_MODEL_WATCHER', true),4 'events' => ['eloquent.created*', 'eloquent.updated*'],5 ],6 // ...7],
如果您想记录给定请求期间水合模型的数量,请启用 hydrations
选项
1'watchers' => [2 Watchers\ModelWatcher::class => [3 'enabled' => env('TELESCOPE_MODEL_WATCHER', true),4 'events' => ['eloquent.created*', 'eloquent.updated*'],5 'hydrations' => true,6 ],7 // ...8],
通知监视器
通知监视器记录应用程序发送的所有通知。 如果通知触发电子邮件并且您启用了邮件监视器,则该电子邮件也将在邮件监视器屏幕上进行预览。
查询监视器
查询监视器记录应用程序执行的所有查询的原始 SQL、绑定和执行时间。 监视器还会将任何慢于 100 毫秒的查询标记为 slow
。 您可以使用监视器的 slow
选项自定义慢查询阈值
1'watchers' => [2 Watchers\QueryWatcher::class => [3 'enabled' => env('TELESCOPE_QUERY_WATCHER', true),4 'slow' => 50,5 ],6 // ...7],
Redis 监视器
Redis 监视器记录应用程序执行的所有 Redis 命令。 如果您正在使用 Redis 进行缓存,缓存命令也将由 Redis 监视器记录。
请求监视器
请求监视器记录与应用程序处理的任何请求关联的请求、标头、会话和响应数据。 您可以通过 size_limit
(以千字节为单位)选项限制记录的响应数据
1'watchers' => [2 Watchers\RequestWatcher::class => [3 'enabled' => env('TELESCOPE_REQUEST_WATCHER', true),4 'size_limit' => env('TELESCOPE_RESPONSE_SIZE_LIMIT', 64),5 ],6 // ...7],
计划任务监视器
计划任务监视器记录应用程序运行的任何计划任务的命令和输出。
视图监视器
视图监视器记录渲染视图时使用的视图名称、路径、数据和“组合器”。
显示用户头像
Telescope 仪表盘显示在保存给定条目时已通过身份验证的用户的用户头像。 默认情况下,Telescope 将使用 Gravatar Web 服务检索头像。 但是,您可以通过在 App\Providers\TelescopeServiceProvider
类中注册回调来自定义头像 URL。 回调将接收用户的 ID 和电子邮件地址,并应返回用户的头像图像 URL
1use App\Models\User; 2use Laravel\Telescope\Telescope; 3 4/** 5 * Register any application services. 6 */ 7public function register(): void 8{ 9 // ...10 11 Telescope::avatar(function (string $id, string $email) {12 return '/avatars/'.User::find($id)->avatar_path;13 });14}